Dynamic visualizations are as simple as a loop
for t in range(100): plot( x=x, y=np.cos(x + t / 10) ) time.sleep(0.01)
Visualize in realtime directly from your Python scripts — no notebooks, servers, or browsers required.
Visualizations are displayed in a dedicated tab right within the IDE.
Integrated plotting toolkits
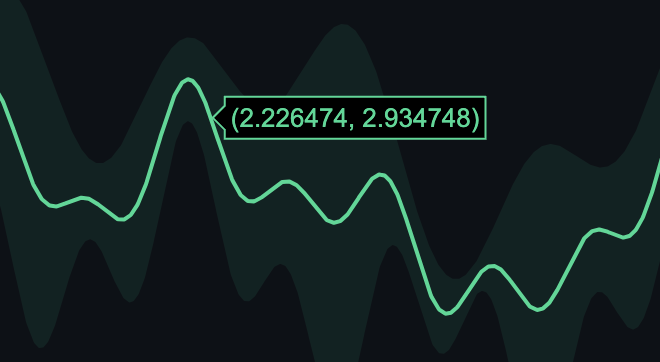
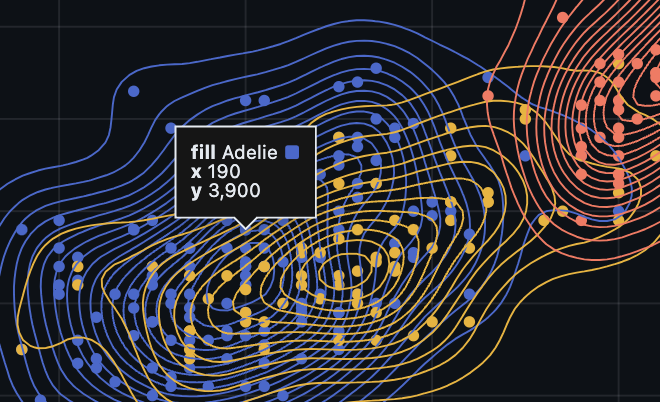
Scripton includes built-in plotting toolkits that expose the capabilities of Plotly and Observable Plot directly to Python.
No installation is necessary — these libraries are automatically available when executing in Scripton.
from scripton.lyra import plot import numpy as np # Evenly spaced values between 0 and 2π x = np.linspace(0, 2 * np.pi) # Plot sin(x) plot({'x': x, 'y': np.sin(x)})
For more details, check out the Scripton plotting documentation for Lyra (toolkit for Plotly) and Orion (toolkit for Observable Plot).
Realtime visualizations for science & engineering
Scripton's architecture combines high-performance interprocess communication for low latency and high throughput on the backend, while leveraging GPU-accelerated rendering to deliver real-time visualizations.
Visualizing matrices (2D NumPy arrays, PyTorch tensors, …) is as simple as this —
import numpy as np from scripton import show x = np.arange(100) toeplitz = np.abs(x[:, None] - x) # Show a colormapped version of the matrix show(toeplitz)
Learn more about visualizing matrices .
Built-in graphics toolkit
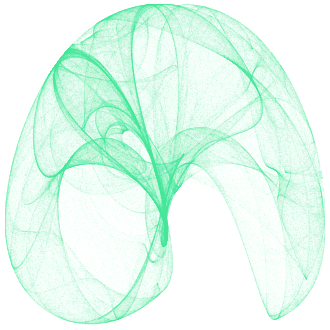
draw_circle
calls — one for each point.
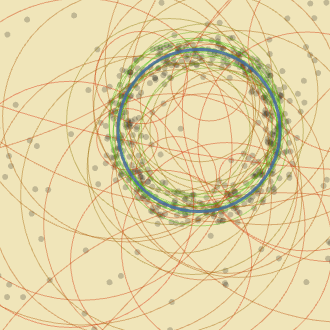
The built-in Scripton canvas library provides a fast and powerful 2D raster graphics API.
from scripton.canvas import Canvas canvas = Canvas(width=290, height=200) for i in range(10): canvas.draw_circle( x=100 + 5 * i, y=100, radius=10 + 10 * i, fill='#3D79FF20' )
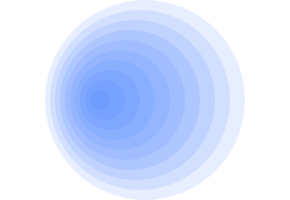
The outputs appear directly in the IDE in realtime in a dedicated tab.
Learn more about Scripton's canvas graphics API.
Rapidly prototype interactivity
render
callback shown in the code snippet, which updates
the visualizations based on the new state. It also returns the updated user interface, which
itself may be dynamic.
Easily add interactivity to your scripts using Scripton's UI toolkit.
from scripton import ui ... def render(state): # Process using current state depth, image = mask_using_depth( threshold=state.threshold ) # Display results show(depth, title='Depth', key='depth') show(image, title='Masked Output', key='image') def reset(): state.threshold = 20 return ui.HStack( ui.Button( label='Reset', on_click=reset ), ui.Slider( label='Depth Threshold', value=state.bind.threshold, range=(0, 20) ) )
Learn more about the UI toolkit.
A rich REPL by your side
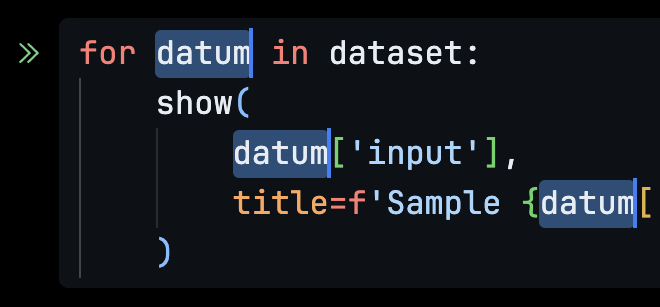
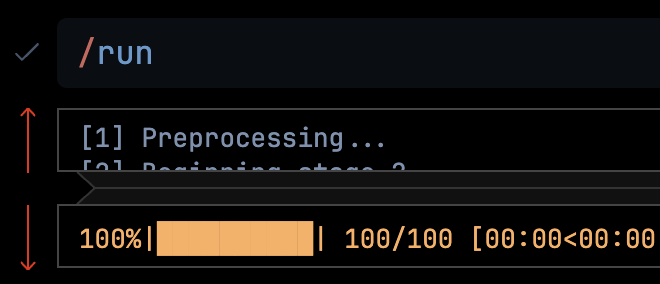
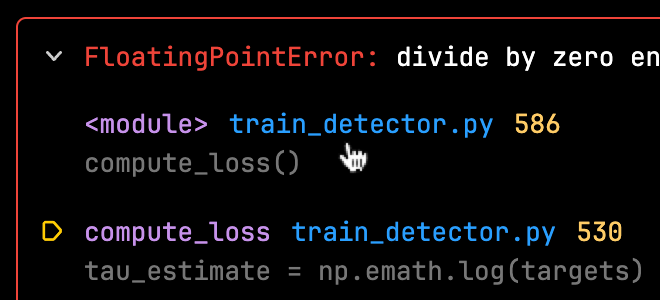
Scripton includes a unique Python REPL that's designed to be a natural extension of your coding environment.
The REPL offers a full-featured editing experience with features like auto-completion, multi-line editing, and multiple cursor support. Outputs can be collapsed to save space, and Python exceptions are syntax-highlighted with clickable navigation to source code. Rich inline outputs are displayed for objects like Pandas data frames and PIL images.
Learn more about the REPL's capabilities.
Frictionless and fast debugging
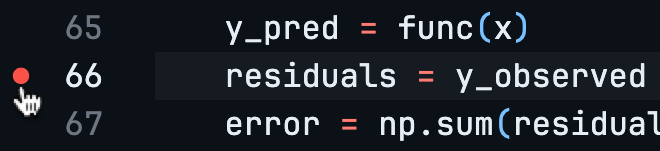

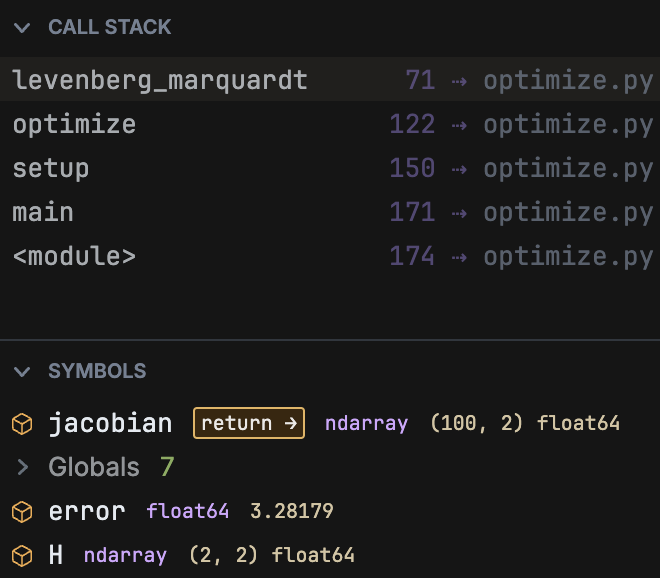
Debugging in Scripton is simple — set a breakpoint and run. No special configurations necessary.
Learn more about debugging in Scripton.
Built-in support for many third party libraries
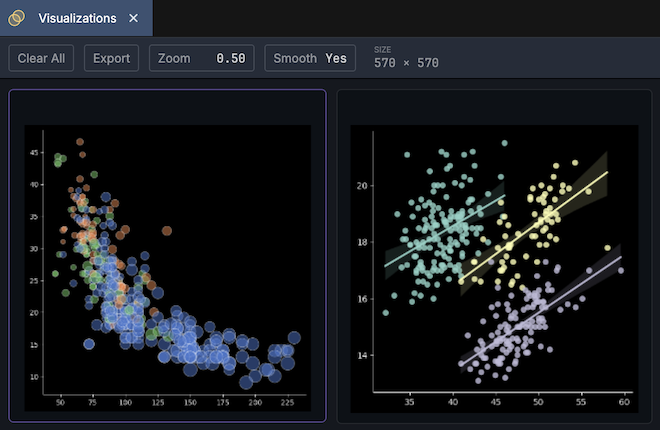
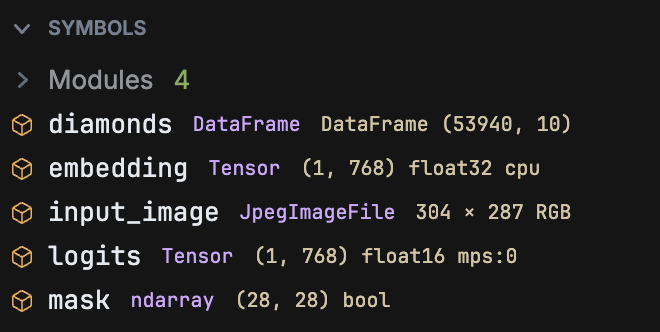
AI features using GitHub Copilot
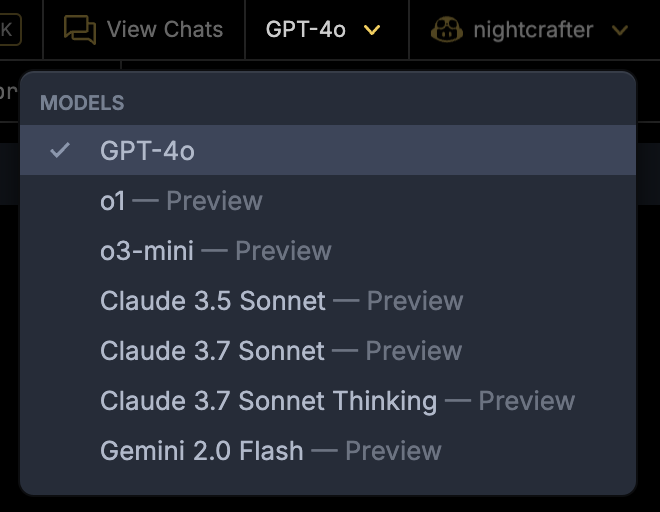
GitHub Copilot support is built into Scripton. You can use your existing GitHub Copilot subscription (including the free plan) to enable the AI features described below.
AI Code Completion
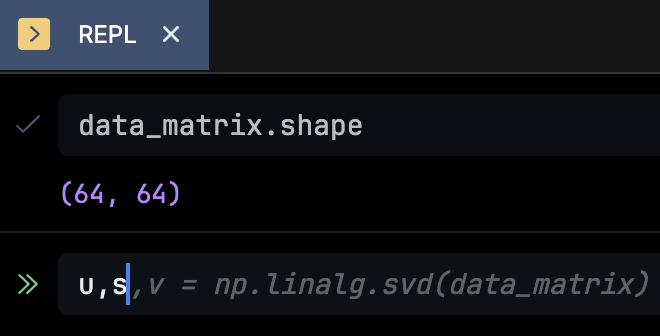
Scripton supports AI powered code completion in the editor as well as the REPL.
The REPL code completion is context aware and capable of taking advantage of past inputs to provide completion suggestions.
AI Code Generation
Directly generate code from within the editor.
The surrounding code is automatically used for context, like the auto-inferred DataFrame
column names
in the example below.
Pair it with Scripton's REPL and visualization tools to rapidly test, iterate, and refine the generated code.
AI Chat
Chat with an AI right from the IDE.
Easily include sources from your workspace as additional context.
Run examples with a single click —
Your conversations are saved privately and locally on your device for easy future reference.
For serious work and fun explorations
canvas
and ui
toolkits.
Scripton's designed and engineered to serve a wide range of needs, from research and industrial applications to creative explorations.
System Requirements
Scripton currently requires macOS (Apple Silicon based models and older Intel based models are both supported). Windows and Linux support is planned.
Python 3.8 or newer is required. Scripton supports a wide range of existing Python environment managers.
Contact
You can reach us at contact@scripton.dev
Scripton includes built in support for many third-party libraries. For instance:
Matplotlib (and libraries based on it, like Seaborn) plots appear within the IDE
PyTorch tensors display their
shape
,dtype
, anddevice
in the symbol explorerPandas DataFrames and PIL images display inline previews in the REPL
OpenCV
imshow
calls are displayed inside the IDE